Assembly part 2.
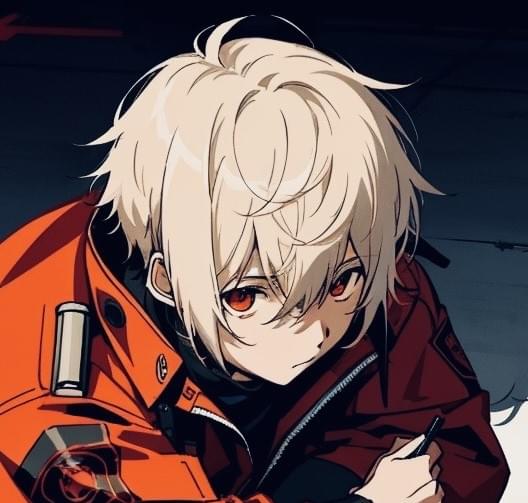
Basic Instructions
1 | ; Program to show use of interrupts |
CMP
CMPX1,X2 JMP
- JE (Jump if Equal): Jumps if the Zero Flag (ZF) is set (
ZF = 1
). - JNE (Jump if Not Equal): Jumps if the Zero Flag (ZF) is not set (
ZF = 0
). - JZ (Jump if Zero): Jumps if the Zero Flag (ZF) is set (
ZF = 1
). - JNZ (Jump if Not Zero): Jumps if the Zero Flag (ZF) is not set (
ZF = 0
). - JS (Jump if Sign): Jumps if the Sign Flag (SF) is set (
SF = 1
). - JNS (Jump if Not Sign): Jumps if the Sign Flag (SF) is not set (
SF = 0
). - JC (Jump if Carry): Jumps if the Carry Flag (CF) is set (
CF = 1
). - JNC (Jump if Not Carry): Jumps if the Carry Flag (CF) is not set (
CF = 0
). - JO (Jump if Overflow): Jumps if the Overflow Flag (OF) is set (
OF = 1
). - JNO (Jump if Not Overflow): Jumps if the Overflow Flag (OF) is not set (
OF = 0
). - JA (Jump if Above): Jumps if (
CF = 0
andZF = 0
). - JAE (Jump if Above or Equal): Jumps if (
CF = 0
). - JNA (Jump if Not Above): Jumps if (
CF = 1
andZF = 1
). - JNAE (Jump if Not Above or Equal): Jumps if (
CF = 1
). - JG (Jump if Greater): Jumps if (
ZF = 0
andSF = OF
). - JGE (Jump if Greater or Equal): Jumps if (
SF = OF
). - JNG (Jump if Not Greater): Jumps if (
ZF = 1
orSF != OF
). - JNGE (Jump if Not Greater or Equal): Jumps if (
SF != OF
). - JB (Jump if Below): Jumps if (
CF = 1
). - JBE (Jump if Below or Equal): Jumps if (
CF = 1
orZF = 1
). - JNB (Jump if Not Below): Jumps if (
CF = 0
). - JNBE (Jump if Not Below or Equal): Jumps if (
CF = 0
andZF = 0
). - JL (Jump if Less): Jumps if (
SF != OF
). - JLE (Jump if Less or Equal): Jumps if (
SF != OF
orZF = 1
). - JNL (Jump if Not Less): Jumps if (
SF = OF
). - JNLE (Jump if Not Less or Equal): Jumps if (
SF = OF
andZF = 0
). - JP/JPE (Jump if Parity/Even Parity): Jumps if Parity Flag (PF) is set (
PF = 1
). - JNP/JPO (Jump if Not Parity/Odd Parity): Jumps if Parity Flag (PF) is not set (
PF = 0
).
Integer Addition and Subtraction
1 | start: |
1 | start: |
1 | start: |
- Operation List
1 | ADD X1,X2 ;將X1 X2的數字相加並存入X1中 |
Exam
- Title: Assembly part 2.
- Author: Chihhh Linnn
- Created at : 2024-08-15 01:24:08
- Updated at : 2024-08-15 01:24:08
- Link: https://chihhhs.github.io/2024/08/15/asm-1/
- License: This work is licensed under CC BY-NC-SA 4.0.