OS Bounded Buffer
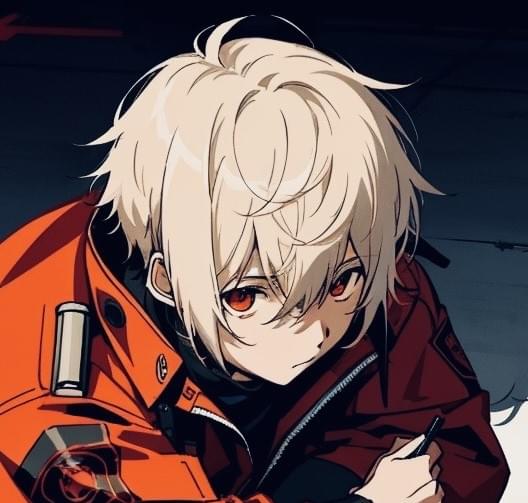
INTRO
共享記憶體的解決方案 (Share memory Solution)
使用一個共享的Buffer(bounded buffer),這個Buffer有固定大小,生產者將數據放入Buffer,消費者從中取出數據。為了保證生產者和消費者不會同時訪問Buffer,使用互斥鎖 (mutex) 和兩個條件變量 (condition variables),一個用於通知Buffer未滿 (not full),另一個用於通知Buffer非空 (not empty)。生產者 (Producer)
生產者的工作是將數據放入Buffer中,當Buffer滿時,它會等待消費者取走一些數據以騰出空間。消費者 (Consumer)
消費者的工作是從Buffer中取出數據,當Buffer為空時,它會等待生產者放入新的數據。Main Funtion
- 初始化 mutex
prod_thread, cons_thread
保存新建 Thread ID , 創建生產者跟消費者 Thread- 等待 Thread 完成
- 釋放它們佔用的資源 (destory)
LIB Function (pthread.h)
IMPL
1 |
|
- Title: OS Bounded Buffer
- Author: Chihhh Linnn
- Created at : 2024-08-26 13:57:36
- Updated at : 2024-08-26 13:57:36
- Link: https://chihhhs.github.io/2024/08/26/os-bbuff/
- License: This work is licensed under CC BY-NC-SA 4.0.